This example demonstrates how to set up the flame sensor with Quarky to detect heat or flame nearby. Later, we create an alarm system triggered with the flame sensor.
Flame Sensor Connection to Quarky
Flame sensors have 4 pins: GND, VCC, DO, and AO. You have to connect the following 3 pins to the Quarky Expansion Board:
- GND to Ground Pin of Quarky Expansion Board
- VCC to 3.3V or VCC Pin of Quarky Expansion Board
- DO to the D3 (Digital Pin) of the Quarky Expansion Board
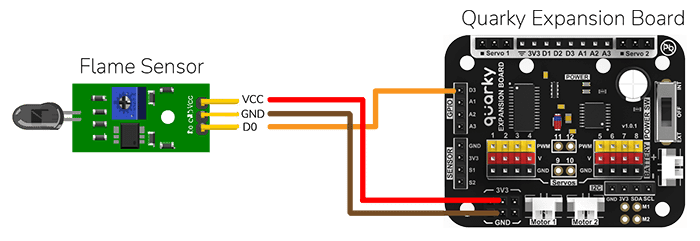
Calibrating Flame Sensor
The sensor also has 2 LEDs – Power and Detection. The desired calibration is achieved when the sensor is inactive when there is no heat or flame nearby and active when the flame is nearby. It is visible on the detection LED.
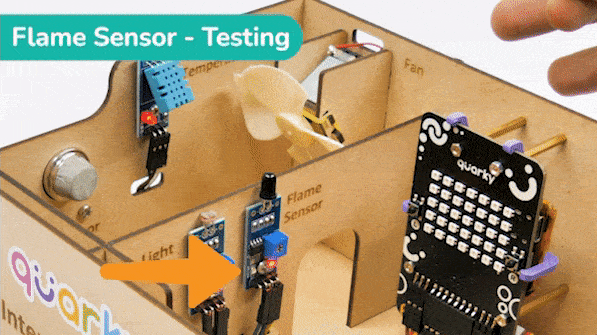
To calibrate the flame sensor:
- Turn the power on for the sensor.
- Place the sensor close to the heat or flame. You should see the detection LED turn on.
- If the LED is Off, adjust the potentiometer until the detection LED turns on.
- Move the sensor away from the heat or flame. The detection LED should turn off.
- If the detection LED does not turn off, continue to adjust the potentiometer until it does.
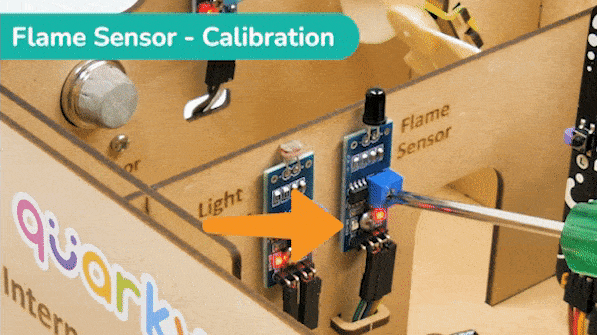
Project: Flame-based Alarm System
In the project, when heat or flame is detected, the alarm system starts with
- The fan turned ON.
- Quakry beeping with lights.
- The door is open for urgent evacuation.
The alarm system will be on until the flame sensor stops detecting the fire.
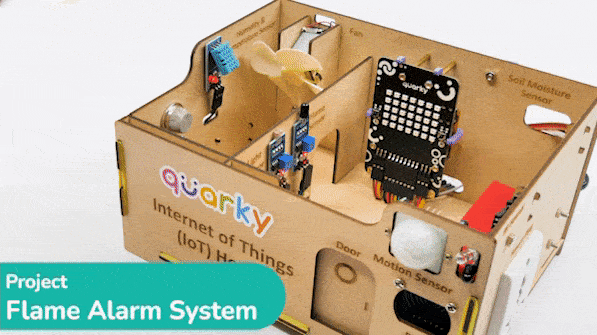
Circuit
Connect the following modules to the Quarky Expansion Board:
- Flame Sensor
- GND to Ground Pin of Quarky Expansion Board
- VCC to 3.3V or VCC Pin of Quarky Expansion Board
- DO to the D3 (Digital Pin) of the Quarky Expansion Board
- Motor Fan: Connect the motor to the Motor Port 1 of the Quarky Expansion Board.
- Door Servo Motor: Connect the servo motor to the servo port 5 of the Quarky Expansion Board.
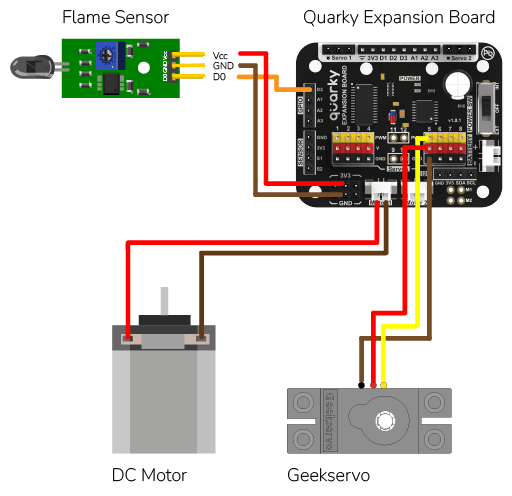
Python Code
- The code first creates objects of the Quarky, Expansion, and IoTHouse classes.
- It then moves the servo connected to pin 5 to 100 degrees and stops the motor connected to pin 1.
- Then it defines two functions, fireDetectedSequence() and fireStopSequence().
- When the fire is detected, it moves the servo connected to pin 5 to 0 degrees, runs the motor connected to pin 1 in clockwise direction with speed 100, plays a tone at C4 pitch for 8 beats, and draws a red pattern on the Quarky display.
- When the fire is no longer detected, it stops the motor connected to pin 1, clears the display of the Quarky robot, and moves the servo connected to pin 5 to 100 degrees.
- In the while loop, the code keeps on checking the flame status of pin D3 in the house.
- If the flame is detected, it waits for two seconds and again checks the flame status. If the flame is still detected, it runs the fireDetectedSequence() and then runs the fireStopSequence().
# The following code is written to detect a fire in a house using a Quarky robot and Expansion board.
# quarky is an instance of the Quarky class which has functionalities like playing a tone and drawing a pattern on LED Screen
quarky = Quarky()
# quarkyexpansion is an instance of the Expansion class which has functionalities like moving a servo and running a motor
quarkyexpansion = Expansion()
# house is an instance of the IoTHouse class which has functionalities like checking the flame status
house = IoTHouse()
# import time library which has functionalities like sleeping for a certain amount of time
import time
# move the servo connected to pin 5 to 100 degrees
quarkyexpansion.moveservo(5, 100)
# stop the motor connected to pin 1
quarkyexpansion.stopmotor(1)
# define a function which initiate instructions when the flame is detected
def fireDetectedSequence():
# move the servo connected to pin 5 to 0 degrees
quarkyexpansion.moveservo(5, 0)
# run the motor connected to pin 1 in clockwise direction with speed 100
quarkyexpansion.runmotor(1, 1, 100)
# keep on checking the flame status of pin D3 in the house, until it is no longer in flame
while not (house.flamestatus("D3")):
# clear the display of the Quarky robot
quarky.cleardisplay()
# play a tone at C4 pitch for 8 beats
quarky.playtone("C4", 8)
# draw red pattern on the Quarky display
quarky.drawpattern("bbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbb")
time.sleep(0.7)
# define a function which is initiated when no flame is detected
def fireStopSequence():
# move the servo connected to pin 5 to 100 degrees
quarkyexpansion.moveservo(5, 100)
# stop the motor connected to pin 1
quarkyexpansion.stopmotor(1)
# clear the display of the Quarky robot
quarky.cleardisplay()
while True:
# keep on checking the flame status of pin D3 in the house
if not (house.flamestatus("D3")):
time.sleep(2)
# again check the flame status of pin D3 in the house
if not (house.flamestatus("D3")):
# if flame is detected, run the fireDetectedSequence()
fireDetectedSequence()
# and then run the fireStopSequence()
fireStopSequence()
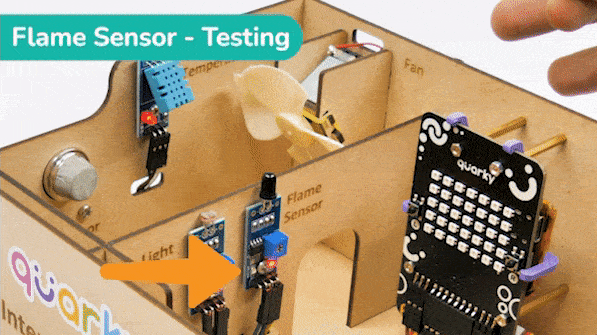
Adding IoT in Fire Alarm System
As an advanced system, we can also send the fire detection alert to the users using IFTTT. For that, we will use IFTTT webhooks.
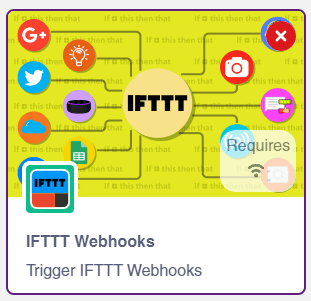
The following IFTTT sequence is to be created:
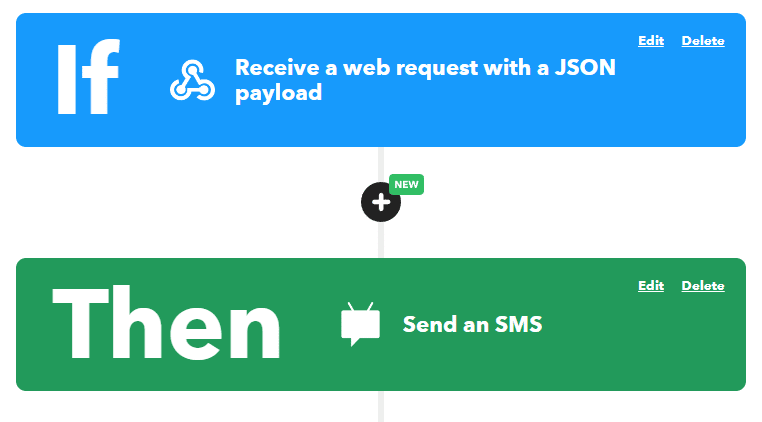
You can learn in detail how to create an IFTTT applet here: https://ai.thestempedia.com/extension/ifttt-webhooks/
Code
This code is the continuation of the past code:
- The code will continuously check the flame status of pin D3 in the house.
- If the flame is detected, it will initiate the fireDetectedSequence() which will send an event to IFTTT Webhooks, move the servo connected to pin 5 to 0 degrees, run the motor connected to pin 1 in the clockwise direction with speed 100, clear the display of the Quarky robot, and play a tone at C4 pitch for 8 beats.
- Once the flame status is no longer detected, it will initiate the fireStopSequence() which will send an event to IFTTT Webhooks, move the servo connected to pin 5 to 100 degrees, stop the motor connected to pin 1, and clear the display of the Quarky robot.
# The following code is written to detect a fire in a house using a Quarky robot and Expansion board.
# quarky is an instance of the Quarky class which has functionalities like playing a tone and drawing a pattern on LED Screen
quarky = Quarky()
# quarkyexpansion is an instance of the Expansion class which has functionalities like moving a servo and running a motor
quarkyexpansion = Expansion()
# house is an instance of the IoTHouse class which has functionalities like checking the flame status
house = IoTHouse()
# import time library which has functionalities like sleeping for a certain amount of time
import time
#Create an instance of the IFTTTWebhooks library
ifttt = IFTTTWebhooks()
# move the servo connected to pin 5 to 100 degrees
quarkyexpansion.moveservo(5, 100)
# stop the motor connected to pin 1
quarkyexpansion.stopmotor(1)
#Set the webhook key and event name
ifttt.setifttt("Flame_Detected", "iNyFg77wDLYV-V9UtdXVtmeebiOw_72LjxZud084ybr")
# define a function which initiate instructions when the flame is detected
def fireDetectedSequence():
#Set the message and priority
ifttt.setvalues("Fire Started! Evacuation Started", 1)
ifttt.triggerevent() #Send the event
# move the servo connected to pin 5 to 0 degrees
quarkyexpansion.moveservo(5, 0)
# run the motor connected to pin 1 in clockwise direction with speed 100
quarkyexpansion.runmotor(1, 1, 100)
# keep on checking the flame status of pin D3 in the house, until it is no longer in flame
while not (house.flamestatus("D3")):
# clear the display of the Quarky robot
quarky.cleardisplay()
# play a tone at C4 pitch for 8 beats
quarky.playtone("C4", 8)
# draw red pattern on the Quarky display
quarky.drawpattern("bbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbbb")
time.sleep(0.7)
# define a function which is initiated when no flame is detected
def fireStopSequence():
#Set the message and priority
ifttt.setvalues("Fire Stopped", 1)
ifttt.triggerevent() #Send the event
# move the servo connected to pin 5 to 100 degrees
quarkyexpansion.moveservo(5, 100)
# stop the motor connected to pin 1
quarkyexpansion.stopmotor(1)
# clear the display of the Quarky robot
quarky.cleardisplay()
while True:
# keep on checking the flame status of pin D3 in the house
if not (house.flamestatus("D3")):
time.sleep(2)
# again check the flame status of pin D3 in the house
if not (house.flamestatus("D3")):
# if flame is detected, run the fireDetectedSequence()
fireDetectedSequence()
# and then run the fireStopSequence()
fireStopSequence()