The function returns the last data value of the specified feed as a string.
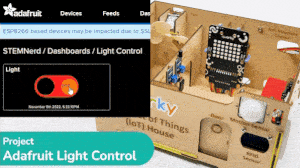
Learn how to create a light switch in Adafruit IO with Python code. Step-by-step guide on how to connect Quarky to Adafruit IO and create a dashboard with a toggle block.
In this example, we are going to understand how to make a feed on Adafruit IO. Later we will write the Python code that will retrieve the information from the cloud and make the Quarky lights ON and OFF.
Adafruit IO Key
You can find the information about your account once you log in from here:
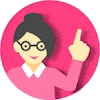
Note: Make sure you are login on Adafruit IO: https://io.adafruit.com/
Creating a Light Switch in Adafruit IO
- Create a new Feed named Light.
- Create a new Dashboard named Light Control.
- Edit the Dashboard and add a Toggle Block.
- Connect the Light feed to the block and click on Next Step.
- Edit the Block Setting and click on Create Block.
- Block is added. You can try to toggle the switch.
- Go to the Light feed. You will observe the value of the feed changing as we click on the switch on the Dashboard.
Python Code for Stage Mode
This Python code creates two instances, one of the Quarky class and one of the AdaIO class. It sets the brightness of the Quarky instance to 10 and connects to Adafruit IO using the specified username and key. It then creates a loop that checks to see if the value of the “Light“ feed from Adafruit IO is “ON“. If the data is “ON” then the white light is turned on on the display, otherwise, the display is cleared.
# Create a new instance of the Quarky class and call it quarky
quarky = Quarky()
# Create a new instance of the AdaIO class and call it adaio
adaio = AdaIO()
# Set the brightness of Quarky to 10
quarky.setbrightness(10)
# Connect to Adafruit IO using the specified username and key
adaio.connecttoadafruitio("STEMNerd", "aio_UZBB56f7VTIDWyIyHX1BCEO1kWEd")
# Create an loop
while True:
# Check to see if the value of the "Light" feed from Adafruit IO is "ON"
if (adaio.getdata("Light") == "ON"):
# If the data is "ON" draw the specified pattern
quarky.drawpattern("aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa")
# Otherwise, clear the display
else:
quarky.cleardisplay()
Output
Python Code for Upload Mode
You can also make the script work independently of PictoBlox using the Upload Mode. For that switch to upload mode.
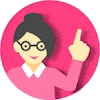
Note: In the upload mode your Quarky needs to connect to the WiFi network. You can learn how to do it here: https://ai.thestempedia.com/example/wi-fi-connect-for-quarky-in-upload-mode-python/
# This code connects to a wifi network and an adafruit IO account.
# It also uses the module "quarky" to set the brightness and draw a pattern.
# The code will check if the wifi is connected and if the value of "Light" in the adafruit IO account is "ON",
# it will draw a pattern on the display. If not, it will clear the display.
# If the wifi is not connected, it will set LED 1 to red.
from quarky import *
# imported module
import iot
# Connect to a wifi network
wifi = iot.wifi()
wifi.connecttowifi("IoT", "12345678")
# Connect to an adafruit IO account
adaio = iot.AdaIO()
adaio.connecttoadafruitio("STEMNerd", "aio_UZBB56f7VTIDWyIyHX1BCEO1kWEd")
# Set the brightness
quarky.setbrightness(10)
while True:
# Check if the wifi is connected
if wifi.iswificonnected():
# Check if the value of "Light" in the adafruit IO account is "ON"
if (adaio.getdata("Light") == "ON"):
# Draw a while LED pattern on the display
quarky.drawpattern("aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa")
else:
# Clear the display
quarky.cleardisplay()
else:
# Set LED 1 to red
quarky.setled(1, 1, [255, 0, 0], 100)
Troubleshooting:
- If the Green Light comes, your Wi-Fi is connected.
- If the Red Light comes, your Wi-Fi is not connected. Change the Wi-Fi and the password and try again.
- If the Red Cross sign comes, Quarky, Python error has occurred. Check the serial monitor and try to reset the Quarky.
Read More