Introduction
A face-tracking robot is a type of robot that uses sensors and algorithms to detect and track human faces in real time. The robot’s sensors, such as cameras or infrared sensors, capture images or videos of the surrounding environment and use computer vision techniques to analyze the data and identify human faces. One of the most fascinating activities is face tracking, in which the Quadruped can detect a face and move its head in the same direction as yours. How intriguing it sounds, so let’s get started with the coding for a face-tracking Quadruped robot.
we will learn how to use face detection to control the movement of a Quadruped robot and how to incorporate external inputs into a program to create more interactive and responsive robotics applications.
Logic
- If the face is tracked at the center of the stage, the Quadruped should be straight.
- As the face moves to the left side, the Quadruped will also move to the left side.
- As the face moves to the right side, the Quadruped will also move to the right side.
Code
sprite = Sprite('Tobi')
quarky=Quarky()
import time
import math
quad=Quadruped(4,1,8,5,3,2,7,6)
fd = FaceDetection()
fd.video("on", 0)
fd.enablebox()
fd.setthreshold(0.5)
time.sleep(1)
Angle=0
while True:
fd.analysestage()
for i in range(fd.count()):
sprite.setx(fd.x(i + 1))
sprite.sety(fd.y(i + 1))
sprite.setsize(fd.width(i + 1))
Angle=fd.width(i + 1)
angle=int(float(Angle))
if angle>90:
quad.move("lateral right",1000,1)
elif angle<90:
quad.move("lateral left",1000,1)
else:
quad.home()
Code Explanation
- First, we import libraries and create objects for the time and math.
- Next, we set up the camera and enable face detection with a 0.5 threshold.
- Based on this information, We use a loop to continuously analyze the camera feed for faces and control the humanoid’s movement.
- When a face is detected, the quadruped sprite moves to the face’s location and the angle of the face is used to determine the direction of movement.
- The Quadruped moves to the left if the angle is greater than 90 degrees.
- The Quadruped moves to the right if the angle is less than 90 degrees.
- If the angle is exactly 90 degrees, the Qudruped returns to its original position.
Output
Our next step is to check whether it is working right or not. Whenever your face will come in front of the camera, it should detect it and as you move to the right or left, the head of your Quadruped robot should also move accordingly.
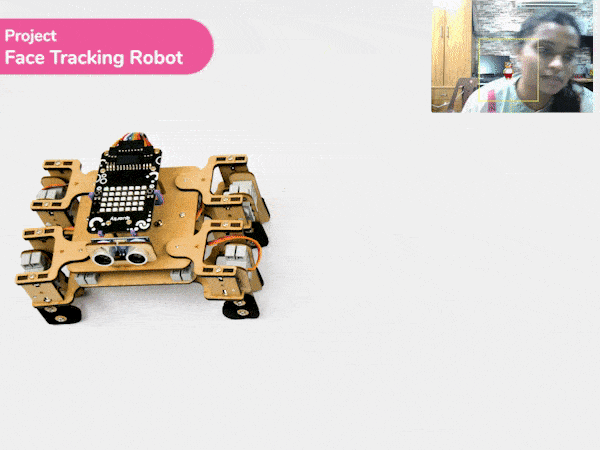