Introduction
One of the most fascinating activities is face tracking, in which the Quarky can detect a face and move its head in the same direction as yours. How intriguing it sounds, so let’s get started with the coding for a face-tracking robot.
Logic
- If the face is tracked at the center of the stage, the humanoid should be straight.
- As the face moves to the left side, the humanoid will also move to the left side.
- As the face moves to the right side, the humanoid will also move to the right side.
Code
sprite = Sprite('Tobi')
quarky=Quarky()
import time
import math
humanoid = Humanoid(7,2,6,3,8,1)
fd = FaceDetection()
fd.video("on", 0)
fd.enablebox()
fd.setthreshold(0.5)
time.sleep(1)
Angle=0
while True:
fd.analysestage()
for i in range(fd.count()):
sprite.setx(fd.x(i + 1))
sprite.sety(fd.y(i + 1))
sprite.setsize(fd.width(i + 1))
Angle=fd.width(i + 1)
angle=int(float(Angle))
if angle>90:
humanoid.move("left",1000,3)
elif angle<90:
humanoid.move("right",1000,3)
time.sleep(1)
else:
humanoid.home()
Code Explanation
- First, we import libraries and create objects for the robot.
- Next, we set up the camera and enable face detection with a 0.5 threshold.
- We use a loop to continuously analyze the camera feed for faces and control the humanoid’s movement based on this information.
- When a face is detected, the humanoid sprite moves to the face’s location, and the angle of the face is used to determine the direction of movement.
- If the angle is greater than 90 degrees, the humanoid moves to the left.if angle is less than 90 degrees, the humanoid moves to the right.if angle is exactly 90 degrees, the humanoid returns to its original position.
- This code demonstrates how to use face detection to control the movement of a humanoid robot and how to incorporate external inputs into a program to create more interactive and responsive robotics applications.
Output
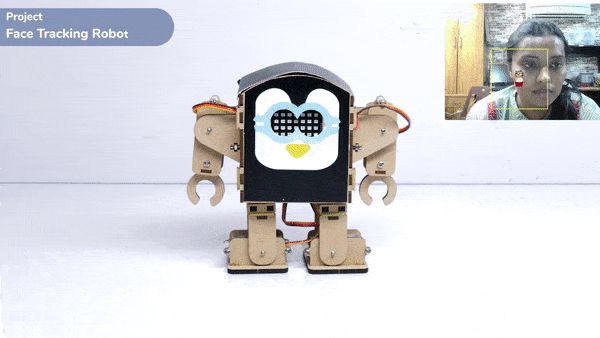