Python math Module
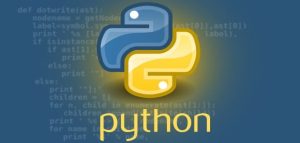
-
Available in: Python Coding
-
Mode: Stage Mode, Upload Mode
-
WiFi Required: No
-
Compatible Hardware in Block Coding: Not Applicable
-
Compatible Hardware in Python: Quarky
-
Object Declaration in Python: import math
-
Extension Catergory: Python Module
Introduction
Python Math Module is a built-in module that contains a large number of mathematical functions. This module is useful for dealing with mathematics-related tasks such as statistics, complex numbers, etc. The math module will also provide useful mathematical constants such as pi.
Definition and Usecase
The math module defines a wide range of useful mathematical functions that can be used directly in any Python program. The use case for Math Module ranges from basic arithmetic operations (such as addition, subtraction, division, etc.), to more complex operations (such as trigonometric functions, complex numbers, etc.).Examples with Output
Examples with Output
Example 1
Below is an example of using the math module to calculate the absolute value of a number.
import math
# initializing the number
number = -2.45
# calculating absolute value of number
absolute_value = math.fabs(number)
# printing the absolute value
print("Absolute value of",number,"is",absolute_value)
Output:
Absolute value of -2.45 is 2.45
Example 2
Below is the example of using math module to calculate the natural logarithm of a number.
import math
# initializing the number
number = 50
# calculating natural log of number
natural_log = math.log(number)
# printing the natural log of number
print("The natural log of", number, "is", natural_log)
Output:
The natural log of 50 is 3.912023005428146