This example demonstrates how to use the Soil Moisture sensor to detect the moisture in the soil and water the plant using the drip system in PictoBlox Python Environment. The system will water the plant when the moisture of the soil is low.
Drip Irrigation Assembly
The following tutorials cover how to make the Drip Irrigation System:
- For 1 Plant: https://ai.thestempedia.com/docs/iot-house-quarky-addon-kit-documentation/1-plant-drip-irrigation-assembly-iot-house/
- For 2 Plants: https://ai.thestempedia.com/docs/iot-house-quarky-addon-kit-documentation/2-plants-drip-irrigation-assembly-iot-house/
Circuit
We are using 2 devices in this project:
- Moisture Sensor: The moisture sensor provides real-time moisture reading from the soil. The moisture sensor connections are as follows:
- GND Pin connected to GND of the Quarky Expansion Board.
- VCC Pin connected to VCC of the Quarky Expansion Board.
- Signal Pin connected to A2 of the Quarky Expansion Board.
- The Water Pump Connected to the Relay: The water pump is controlled by the smart switch of the IoT house which has a relay controlling the state. If the relay is ON, the smart switch gets ON, turning on the water pump. The relay has the following connections:
- GND Pin connected to GND of the Quarky Expansion Board.
- VCC Pin connected to VCC of the Quarky Expansion Board.
- Signal Pin connected to Servo 8 of the Quarky Expansion Board.
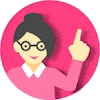
Note: Once the connection is done, make sure you have the drip irrigation system placed with some water in the tank.
Python Code for Stage Mode
The code does the following:
- This code creates two instances, one of the Quarky class called ‘quarky’ and one of the IoTHouse class called ‘house’. It also imports the time library which contains functions for working with time.
- The code then creates two functions.
- The first, Display_Soil_Moisture_Level, uses the Quarky and IoTHouse instances to read the soil moisture level from the IoTHouse and then display the moisture level on the Quarky.
- The second, Watering_Animation, uses the Quarky instance to display a watering animation on the Quarky.
- Finally, the code sets the moisture pin on the IoTHouse to A2 and enters an infinite loop. The loop calls the Display_Soil_Moisture_Level function, and if the moisture level is below 30, it turns on the relay on the IoTHouse and calls the Watering_Animation function until the moisture level is above 80. Once the moisture level is above 80, the loop turns off the relay 0 on the IoTHouse and pauses for 2 seconds before repeating.
# Create an instance of the Quarky class called quarky
quarky = Quarky()
# Create an instance of the IoTHouse class called house
house = IoTHouse()
# Import the time library which contains functions for working with time
import time
# Create a function called Display_Soil_Moisture_Level which will display the soil moisture level on the Quarky
def Display_Soil_Moisture_Level():
# Clear the display on Quarky
quarky.cleardisplay()
# Read the moisture level from the IoTHouse
Moisture = house.readmoisture()
# Iterate through each row and column of the display
for row in range(1, 6):
for column in range(1, 8):
# If the current row and column number is less than the moisture level
# Turn on the corresponding LED on Quarky
if ((((row - 1) * 20) + (column * (10 / 7))) < Moisture):
quarky.setled(column, row, [0, 255, 0], 33)
# Create a function called Watering_Animation to display a watering animation on the Quarky
def Watering_Animation():
# Draw the first pattern on Quarky
quarky.drawpattern("jfjjjfjjdjjjjjfdfjjjjjfjjjjjjjjjjjj")
time.sleep(0.2)
# Draw the second pattern on Quarky
quarky.drawpattern("jjjjfdfjfjjjfjjdjjjjjfdfjjjjjfjjjjj")
time.sleep(0.2)
# Draw the third pattern on Quarky
quarky.drawpattern("jjjjjdjjjjjfdfjfjjjfjjdjjjjjfdfjjjj")
time.sleep(0.2)
# Draw the fourth pattern on Quarky
quarky.drawpattern("jjjjjfjjjjjjdjjjjjfdfjfjjjfjjdjjjjj")
time.sleep(0.2)
# Draw the fifth pattern on Quarky
quarky.drawpattern("jjjjjjjjjjjjfjjjjjjdjjjjjfdfjfjjjfj")
time.sleep(0.2)
# Draw the sixth pattern on Quarky
quarky.drawpattern("jfjjjjjjjjjjjjjjjjjfjjjjjjdjjjjjfdf")
time.sleep(0.2)
# Draw the seventh pattern on Quarky
quarky.drawpattern("fdfjjjjjfjjjjjjjjjjjjjjjjjfjjjjjjdj")
time.sleep(0.2)
# Draw the eighth pattern on Quarky
quarky.drawpattern("jdjjjjjfdfjjjjjfjjjjjjjjjjjjjjjjjfj")
time.sleep(0.2)
# Set the moisture pin on the IoT House to A2
house.setmoisturepin("A2")
# Create an infinite loop
while True:
# Call the Display_Soil_Moisture_Level function
Display_Soil_Moisture_Level()
# If the moisture level is below 30
if (house.readmoisture() < 30):
# Turn on the relay 0 on the IoTHouse
house.setrelay(0, "pwm4")
# Create a loop which will run until the moisture level is above 80
while not ((house.readmoisture() > 80)):
# Call the Watering_Animation function twice
Watering_Animation()
# Turn off the relay 0 on the IoTHouse
house.setrelay(1, "pwm4")
# Pause for 2 seconds
time.sleep(2)
Output
Python Code for Upload Mode
You can also make the script work independently of PictoBlox using the Upload Mode. For that switch to upload mode and replace the when green flag clicked block with when Quarky starts up the block.
Only the object initialization changes in the Upload Mode.
# Create an instance of the Quarky class called quarky
from quarky import *
# Create an instance of the IoTHouse class called house
import iothouse
house = iothouse.iothouse()
# Import the time library which contains functions for working with time
import time
# Create a function called Display_Soil_Moisture_Level which will display the soil moisture level on the Quarky
def Display_Soil_Moisture_Level():
# Clear the display on Quarky
quarky.cleardisplay()
# Read the moisture level from the IoTHouse
Moisture = house.readmoisture()
# Iterate through each row and column of the display
for row in range(1, 6):
for column in range(1, 8):
# If the current row and column number is less than the moisture level
# Turn on the corresponding LED on Quarky
if ((((row - 1) * 20) + (column * (10 / 7))) < Moisture):
quarky.setled(column, row, [0, 255, 0], 33)
# Create a function called Watering_Animation to display a watering animation on the Quarky
def Watering_Animation():
# Draw the first pattern on Quarky
quarky.drawpattern("jfjjjfjjdjjjjjfdfjjjjjfjjjjjjjjjjjj")
time.sleep(0.2)
# Draw the second pattern on Quarky
quarky.drawpattern("jjjjfdfjfjjjfjjdjjjjjfdfjjjjjfjjjjj")
time.sleep(0.2)
# Draw the third pattern on Quarky
quarky.drawpattern("jjjjjdjjjjjfdfjfjjjfjjdjjjjjfdfjjjj")
time.sleep(0.2)
# Draw the fourth pattern on Quarky
quarky.drawpattern("jjjjjfjjjjjjdjjjjjfdfjfjjjfjjdjjjjj")
time.sleep(0.2)
# Draw the fifth pattern on Quarky
quarky.drawpattern("jjjjjjjjjjjjfjjjjjjdjjjjjfdfjfjjjfj")
time.sleep(0.2)
# Draw the sixth pattern on Quarky
quarky.drawpattern("jfjjjjjjjjjjjjjjjjjfjjjjjjdjjjjjfdf")
time.sleep(0.2)
# Draw the seventh pattern on Quarky
quarky.drawpattern("fdfjjjjjfjjjjjjjjjjjjjjjjjfjjjjjjdj")
time.sleep(0.2)
# Draw the eighth pattern on Quarky
quarky.drawpattern("jdjjjjjfdfjjjjjfjjjjjjjjjjjjjjjjjfj")
time.sleep(0.2)
# Set the moisture pin on the IoT House to A2
house.setmoisturepin("A2")
# Create an infinite loop
while True:
# Call the Display_Soil_Moisture_Level function
Display_Soil_Moisture_Level()
# If the moisture level is below 30
if (house.readmoisture() < 30):
# Turn on the relay 0 on the IoTHouse
house.setrelay(0, "pwm4")
# Create a loop which will run until the moisture level is above 80
while not ((house.readmoisture() > 80)):
# Call the Watering_Animation function twice
Watering_Animation()
# Turn off the relay 0 on the IoTHouse
house.setrelay(1, "pwm4")
# Pause for 2 seconds
time.sleep(2)