Introduction
A relational operator is an operator that tests a relation between two entities. The result of a relational operator is either true or false.
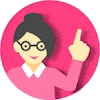
There are 6 relational operators in Arduino IDE:
Relational Operators | Operator Symbol | Example |
---|---|---|
Greater than | > | currentYear > 2015 |
Less than | < | 2015 < currentYear |
Greater than or equal to | >= | currentYear >= 2017 |
Less than or equal to | <= | 2017 <= currentYear |
Equal to | == | centuryYear == 2000 |
Not equal to | != | centuryYear != 1999 |
Greater than (>)
It results in true only if the first number is greater than the second number. If the first number is equal to or less than the second, it results in a false. Given below is an example:
int Result1;
int Result2;
void setup() {
Serial.begin(9600);
Result1 = (7 > 4);
Result2 = (7 > 10);
Serial.print("Is 7 greater than 4: ");
Serial.println(Result1);
Serial.print("Is 7 greater than 10: ");
Serial.println(Result2);
}
void loop() {
}
The result of the operation will be:
Is 7 greater than 4: 1
Is 7 greater than 10: 0
Less than (<)
It results in true only if the first number is less than the second number. If the first number is equal to or greater than the second, it results in a false. Given below is an example:
int Result1;
int Result2;
void setup() {
Serial.begin(9600);
Result1 = (7 < 4);
Result2 = (7 < 10);
Serial.print("Is 7 less than 4: ");
Serial.println(Result1);
Serial.print("Is 7 less than 10: ");
Serial.println(Result2);
}
void loop() {
}
The result of the operation is:
Is 7 less than 4: 0
Is 7 less than 10: 1
Greater than or equal to (>=)
It results in true if the first number is either greater than or equal to the second number. If the first number is less than the second, it results in a false. Given below is an example:
void setup() {
Serial.begin(9600);
Result1 = (7 >= 10);
Result2 = (7 >= 7);
Serial.print("Is 7 greater than or equal to 10: ");
Serial.println(Result1);
Serial.print("Is 7 greater than or equal to 7: ");
Serial.println(Result2);
}
void loop() {
}
The result of the operation is:
Is 7 greater than or equal to 10: 0
Is 7 greater than or equal to 7: 1
Less than or equal to (<=)
It results in true if the first number is either less than or equal to the second number. If the first number is greater than the second, it results in a false. Given below is an example:
int Result1;
int Result2;
void setup() {
Serial.begin(9600);
Result1 = (7 <= 4);
Result2 = (7 <= 7);
Serial.print("Is 7 less than or equal to 4: ");
Serial.println(Result1);
Serial.print("Is 7 less than or equal to 7: ");
Serial.println(Result2);
}
void loop() {
}
The result of the operation is:
Is 7 less than or equal to 4: 0
Is 7 less than or equal 7: 1
Equal to (==)
It results in true only if the first number is equal to the second number; otherwise, it results in false. Given below is an example:
int Result1;
int Result2;
void setup() {
Serial.begin(9600);
Result1 = (7 == 10);
Result2 = (7 == 7);
Serial.print("Is 7 equal to 10: ");
Serial.println(Result1);
Serial.print("Is 7 equal to 7: ");
Serial.println(Result2);
}
void loop() {
}
The result of the operation is:
Is 7 equal to 10: 0
Is 7 equal to 7: 1
Not equal to (!=)
It results in true if the first number is not equal to the second number; otherwise, it results in false. Given below is an example:
int Result1;
int Result2;
void setup() {
Serial.begin(9600);
Result1 = (7 != 10);
Result2 = (7 != 7);
Serial.print("Is 7 not equal to 10: ");
Serial.println(Result1);
Serial.print("Is 7 not equal to 7: ");
Serial.println(Result2);
}
void loop() {
}
The result of the operation is:
Is 7 not equal to 10: 1
Is 7 not equal to 7: 1
Conclusion
In this lesson, we discussed the six relational operators available in the Arduino IDE. We can use these operators to compare two values and get a boolean (true or false) result. We also looked at examples of how to use each operator. With this knowledge, you can now use relational operators in your own projects.