Introduction
The Data Logger module allows you to save data in form of CSV file. You can create and configure file according to your needs and monitor them while they are being logged. This module also works in the background allowing you to save your data while using other modules. Once the data logging process is complete you can also open and share the file. You can also access previously logged files anytime.
For creating a Data Logger file you need to give the following instructions from hardware:
- File name – The name with which you want to generate your CSV.
- Column Name – The name of various columns that you have to create in your data logging sheet.
- Data – Values that are to be stored in various created columns
- Close file – Give this command when you want to stop logging and close the file.
The following images show what the process of data logging looks like in the module:
- A data logging file is created. By using the button marked ‘X’ you can close the created file from the smartphone itself.
- When the process of data logging is going on.
There is an icon beside the “connect” icon it is to open previously made files. You can view these files, share them and also delete the unwanted files.
Arduino IDE Functions
Header
To include the GamePad module in the Arduino program, you have to include the following header:
#define CUSTOM_SETTINGS
#define INCLUDE_DATALOGGER_MODULE
After defining the above mention headers, you have to include the dabble library:
#include <Dabble.h>
You can download the zip of Arduino Library for
- evive, Uno, Mega, and Nano – Dabble-Arduino
- ESP32 – Dabble-ESP32
depending on the board you are using.
Enabling Bluetooth Communication
To enable Bluetooth communication, you have to initialize serial communication using the following code:
- For evive and Arduino Mega, Uno, and Nano
Dabble.begin(Baud_Rate);
Here Baud_Rate is the baud rate set for the Bluetooth module. With evive, you normally get 115200 baud rate modules. - For ESP32
Dabble.begin("Bluetooth_Name");
In place of Bluetooth_Name enter the name that you want to keep for Bluetooth of ESP32. The default name given in the library is “MyEsp32”.
Refreshing the data
To refresh the data that the device has got from the mobile app, you have to use the following line of code:
Dabble.processInput();
Functions
DataLogger.createFile(FileName) – Open a CSV file of name passed as parameter in this function.
DataLogger.createColumn(CoulmnName) – Create column of the name passed as parameter in this function. The previous function created a file. Now in order to create a column in that file, we have to use this function.
DataLogger.send(ColumnName, Data) – As there can be multiple columns in a file hence in order to instruct in which column you want to log data you have to pass that column’s name and the associated data as the parameter.
DataLogger.close() – To close the ongoing file.
DataLogger.sendSettings(&FunctionName) – This is a callback function. It is used to send commands as soon as the Bluetooth connection is established. Its use will be clear from the example.
PictoBlox (Scratch) Blocks
The stacked block “create () named ()” is for configuring the initial parameters of the data logger file. Before starting the logging process you have to create the file in which you will store data. Hence you have to give the file name and the column names in which you will be logging different data values. This block consists of two elements in its drop-down. One is a File and one is Column. The text written in the text field of the block is assigned to either of the element selected from the drop-down.
The “log () with data ()” block is used for sending data that is to be logged in the file. The block has two text fields. In the first text field, you have to write the column name and in the second text field, you have to log the data to be stored. The column name written in the name of any of the columns that you have given while creating columns in your data logger file using the above block.
In order to stop the data logging process and close the currently open data logger file use this block.
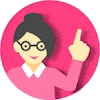
Examples
Arduino IDE
evive
/*
Data Logger module helps you in storing data in form of .csv file.
Later you can open this file to view your stored data.
You can reduce the size of library compiled by enabling only those modules that you want to
use.For this first define CUSTOM_SETTINGS followed by defining INCLUDE_modulename.
Explore more on: https://thestempedia.com/docs/dabble/
*/
#define CUSTOM_SETTINGS
#define INCLUDE_DATALOGGER_MODULE
#include <evive.h>
#include <Dabble.h>
bool isFileOpen = true;
void initializeFile()
{
DataLogger.createFile("Potentiometer3 Data"); //create file (This is a mandatory step)
DataLogger.createColumn("POT-1"); //enter column names (This is a mandatory step)
DataLogger.createColumn("POT-2");
}
void setup() {
Serial.begin(250000); //change baud rate of serial monitor to this baud
Dabble.begin(115200); //enter baudrate of your bluetooth
pinMode(SLIDESW1_D1,INPUT);
pinMode(TACTILESW1,INPUT);
DataLogger.sendSettings(&initializeFile); //callback function executed when bluetooth is connected
}
void loop() {
Dabble.processInput(); //this function is used to refresh data obtained from smartphone.Hence calling this function is mandatory in order to get data properly from your mobile.
if ((digitalRead(SLIDESW1_D1) == HIGH) && isFileOpen == true) //Move Slide Switch up to start data logging.
{
DataLogger.send("POT-1", analogRead(POT1)); //format to send data is to enter (column name,data)
DataLogger.send("POT-2", analogRead(POT2));
//delay(20);
}
if((digitalRead(TACTILESW1) == HIGH) && isFileOpen == true) //press tactile switch once to close current data logger file
{
DataLogger.close(); //to close file created
isFileOpen = false;
}
}
Mega
/*
Data Logger module helps you in storing data in form of .csv file.
Later you can open this file to view your stored data.
You can reduce the size of library compiled by enabling only those modules that you want to
use.For this first define CUSTOM_SETTINGS followed by defining INCLUDE_modulename.
Explore more on: https://thestempedia.com/docs/dabble/
*/
#define CUSTOM_SETTINGS
#define INCLUDE_DATALOGGER_MODULE
#include <Dabble.h>
bool isFileOpen = true;
int startLoggingButton = 2;
int stopLoggingButton = 3;
void initializeFile()
{
DataLogger.createFile("Analog Pin Data"); //create file (This is a mandatory step)
DataLogger.createColumn("Pin-A0"); //enter column names (This is a mandatory step)
DataLogger.createColumn("Pin-A1");
}
void setup() {
Serial3.begin(115200); //enter baudrate of your bluetooth
Dabble.begin(Serial3);
pinMode(startLoggingButton,INPUT_PULLUP);
pinMode(stopLoggingButton,INPUT_PULLUP);
DataLogger.sendSettings(&initializeFile); //callback function executed when bluetooth is connected
}
void loop() {
Dabble.processInput(); //this function is used to refresh data obtained from smartphone.Hence calling this function is mandatory in order to get data properly from your mobile.
if ((digitalRead(startLoggingButton) == LOW) && isFileOpen == true) //Move Slide Switch up to start data logging.
{
DataLogger.send("Pin-A0", analogRead(A0)); //format to send data is to enter (column name,data)
DataLogger.send("Pin-A1", analogRead(A1));
//delay(20);
}
if((digitalRead(stopLoggingButton) == LOW) && isFileOpen == true) //press tactile switch once to close current data logger file
{
DataLogger.close(); //to close file created
isFileOpen = false;
}
}
ESP32
/*
Data Logger module helps you in storing data in form of .csv file.
Later you can open this file to view your stored data.
You can reduce the size of library compiled by enabling only those modules that you want to
use.For this first define CUSTOM_SETTINGS followed by defining INCLUDE_modulename.
Explore more on: https://thestempedia.com/docs/dabble/
*/
#define CUSTOM_SETTINGS
#define INCLUDE_SENSOR_MODULE
#define INCLUDE_DATALOGGER_MODULE
#include <DabbleESP32.h>
bool isFileOpen = true;
uint8_t closeFileSignalPin = 2; //this pin is internally pulled up and a push button grounded on one side is connected to pin so that pin detects low logic when push button is pressed.
void initializeFile(){
Serial.println("Initialize");
DataLogger.createFile("Microphone");
DataLogger.createColumn("Decibel");
}
void setup() {
pinMode(closeFileSignalPin,INPUT_PULLUP);
Serial.begin(115200); // make sure your Serial Monitor is also set at this baud rate.
Dabble.begin("Myesp32"); //set bluetooth name of your device
DataLogger.sendSettings(&initializeFile);
}
void loop() {
Dabble.processInput(); //this function is used to refresh data obtained from smartphone.Hence calling this function is mandatory in order to get data properly from your mobile.
if( isFileOpen == true)
{
print_Sound_data();
DataLogger.send("Decibel",Sensor.getdata_Sound());
}
if((digitalRead(closeFileSignalPin) == LOW) && isFileOpen == true)
{
isFileOpen = false;
DataLogger.stop();
}
}
void print_Sound_data()
{
Serial.print("SOUND: ");
Serial.println(Sensor.getdata_Sound(), 3);
Serial.println();
}